OpenID for ASP.NET Core
Fully compliant with the OpenID Connect specifications
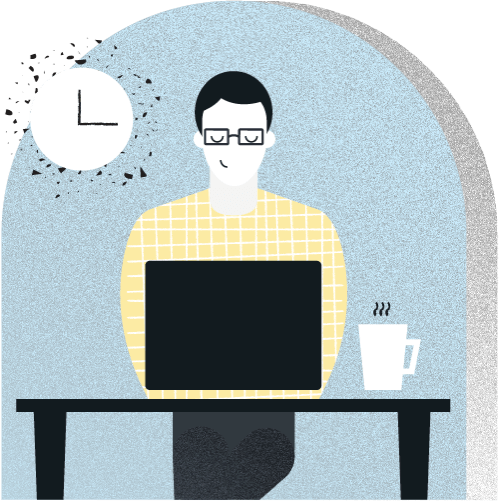
Product Details
The OpenID Connect (OIDC) library for ASP.NET Core allows you to quickly and securely add OpenID Provider support to your application.
Successfully run against the OpenID Foundation's conformance tests (over 450 tests).
Includes Blazor WASM and ASP.NET Core example client projects.
Compatible with all OpenID Connect compliant clients.
Related Products
We also offer a SAML for ASP.NET Core library.
The combination of the OpenID Connect and SAML libraries enables OpenID Connect clients to participate in SSO with SAML identity providers.
OpenID for ASP.NET Core
What's included?
OIDC C# Example Projects to Help You
OpenID Connect ASP.NET Core projects written in C#, with full source code, are included.
The examples demonstrate:
- Acting as the OpenID provider
- Acting as the authorization server
- Supporting metadata requests
- Supporting key requests
- Authentication
- ID token and user information retrieval
- JWT access token generation
- JWT bearer token authorization
- Refresh tokens
- Logout
High Level OIDC API
Add OpenID provider functionality to your web application with just a few lines of code.
A high-level, configuration-driven OIDC API hides the complexities of OpenID Connect, making for a quick and easy implementation.
Configuration changes, including adding support for additional clients, can be made with zero code updates.
Processing an OpenID Discovery Metadata Request
OpenID metadata is returned to the client.
[Route(".well-known/openid-configuration")]
[ResponseCache(Duration = 600, Location = ResponseCacheLocation.Any)]
public async Task GetMetadataAsync()
{
// Return the OpenID provider's metadata.
return await _openIDProvider.GetMetadataAsync();
}
Processing an OpenID Key Request
OpenID keys are returned to the client.
[Route("openid/keys")]
[ResponseCache(Duration = 600, Location = ResponseCacheLocation.Any)]
public async Task GetKeysAsync()
{
// Return the OpenID provider's keys.
return await _openIDProvider.GetKeysAsync();
}
Processing an OpenID Connect Authentication Request
The authorization request is received and processed.
[Route("openid/authorize")]
public async Task AuthorizeAsync()
{
// Receive and process the OpenID Connect authentication request.
var authenticationRequest = await _openIDProvider.ReceiveAuthnRequestAsync();
}
Sending an OpenID Connect Authentication Response
The authorization response is created and sent.
[Route("openid/AuthenticationCompletion")]
public async Task AuthenticationCompletionAsync()
{
// Create and send the OpenID Connect authentication response.
return await _openIDProvider.SendAuthnResponseAsync(name, claims, accessToken);
}
Interoperability
ASP.NET Core example projects are included demonstrating interoperability with:
Documentation
Comprehensive documentation is available to guide you through implementation.
Platform Support
The OIDC library supports ASP.NET Core 6.0 and above. All ASP.NET Core platforms, including Windows, Linux and macOS, are supported.
Source Code
For your assurance and convenience, the complete source code for the OIDC library is available for purchase.
Consulting Inquiries
For consulting and implementation inquires, feel free to contact us at [email protected].
OpenID Connect Specification Compliance
All OpenID Connect flows are supported.
- Authorization Code Flow
- Authorization Code Flow with Proof Key for Code Exchange (PKCE)
- Implicit Flow
- Hybrid Flow
All OpenID Connect response types are supported.
- code
- id_token
- id_token token
- code id_token
- code token
- code id_token token
Supported OpenID Connect client authentication methods:
- client_secret_basic
- client_secret_post
- client_secret_jwt
- private_key_jwt
- none
Supported signature algorithms:
- HS256, HS384, HS512
- RS256, RS384, RS512
- ES256, ES384, ES512
- PS256, PS384, PS512
Supported encryption algorithms (alg):
- A128KW, A192KW, A256KW
- dir
- RSA1_5
- RSA-OAEP
Supported encryption algorithms (enc):
- A128CBC-HS256, A192CBC-HS384, A256CBC-HS512
Successfully tested against the OpenID Foundation's test plans.
- oidcc-config-certification-test-plan
- oidcc-basic-certification-test-plan
- oidcc-implicit-certification-test-plan
- oidcc-hybrid-certification-test-plan
- oidcc-formpost-basic-certification-test-plan
- oidcc-formpost-implicit-certification-test-plan
- oidcc-formpost-hybrid-certification-test-plan
- oidcc-rp-initiated-logout-certification-test-plan (all response types)